Group A
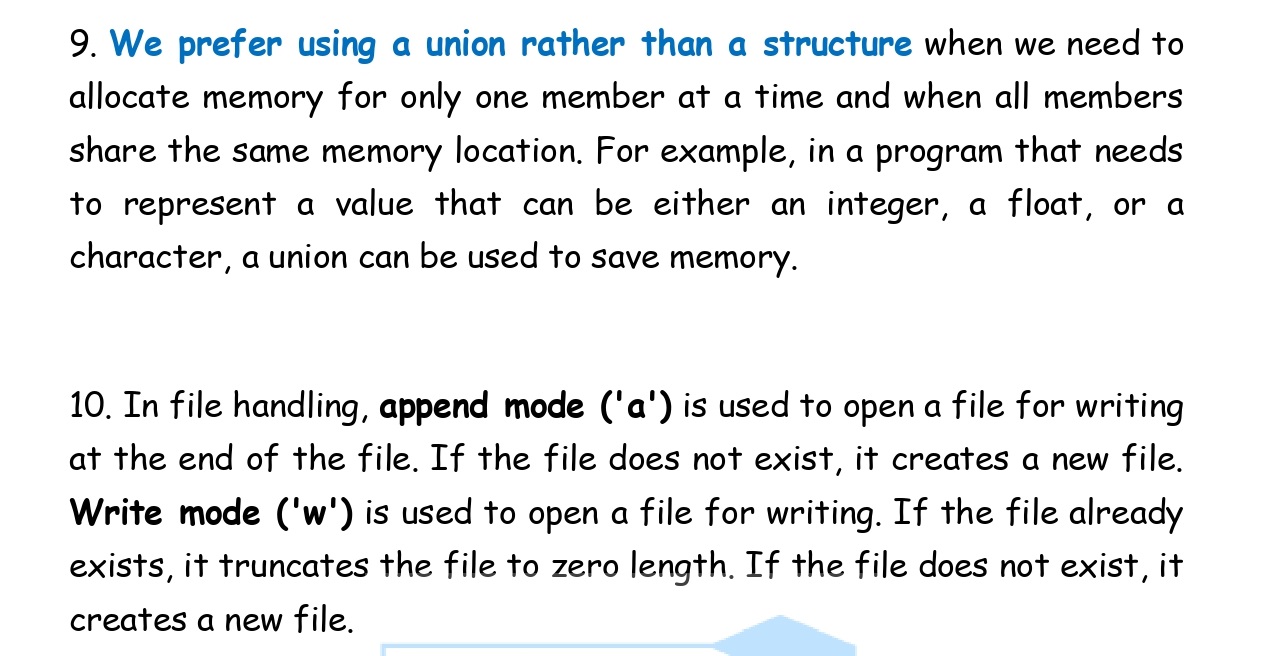
Group B
11.
12.
Data types in C programming include int, float, double, char, and more. They are used to define the type of data that a variable can store and determine the operations that can be performed on that data.
In C programming, there are several data types, including:
- int: Used for integer values.
- float: Used for floating-point numbers.
- double: Used for double-precision floating-point numbers.
- char: Used for single characters.
- void: Represents absence of type.
- _Bool: Represents boolean values (0 for false, any non-zero value for true).
- Enumeration (enum): Used to define custom data types with a finite set of values.
- Array: Used to store a collection of elements of the same data type.
- Pointer: Used to store memory addresses.
Each data type has its specific use case and range of values it can hold.
13.
- Escape sequences: They are special characters in strings preceded by a backslash (
\
). They are used to represent characters that are difficult or impossible to represent directly in code. For example,\n
represents a newline character. - Delimiter: A delimiter is a character used to specify the boundary between separate, independent regions in plain text or other data streams. For example, in CSV files, commas are used as delimiters to separate fields.
Example:
1 2 3 4 5 6 7 8 9 10 |
#include <stdio.h> int main() { // Escape sequence example printf("This is a newline: \n"); // Delimiter example printf("Item 1, Item 2, Item 3\n"); return 0; } |
14.
Pointers in C can be used in arithmetic operations such as addition, subtraction, etc. This is often used to navigate through arrays or allocate memory dynamically.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
#include <stdio.h> int main() { int arr[5] = {1, 2, 3, 4, 5}; int *ptr = arr; // Pointer to the first element of the array // Adding 2 to the pointer moves it to the third element ptr += 2; printf("Third element: %d\n", *ptr); // Output: 3 return 0; } |
15.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
#include <stdio.h> struct mobile { char model[50]; int price; }; int main() { struct mobile phones[3] = { {"iPhone", 80000}, {"Samsung", 12000}, {"OnePlus", 35000} }; for (int i = 0; i < 3; i++) { if (phones[i].price > 10000) { printf("Model: %s\n", phones[i].model); } } return 0; } |
16.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
#include <stdio.h> int main() { FILE *source, *target; char ch; source = fopen("source.txt", "r"); target = fopen("target.txt", "w"); if (source == NULL || target == NULL) { printf("Error in file handling!\n"); return 1; } while ((ch = fgetc(source)) != EOF) { fputc(ch, target); } printf("File copied successfully.\n"); fclose(source); fclose(target); return 0; } |
Group C
17.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
#include <stdio.h> int sumOfDigits(int num) { if (num == 0) { return 0; } else { return (num % 10) + sumOfDigits(num / 10); } } int main() { int num, sum; printf("Enter an integer: "); scanf("%d", &num); sum = sumOfDigits(num); printf("Sum of digits of %d = %d\n", num, sum); return 0; } |
18.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
#include <stdio.h> #include <graphics.h> int main() { int gd = DETECT, gm; initgraph(&gd, &gm, ""); // Coordinates of top-left and bottom-right corners of the rectangle int left = 100, top = 100; int right = 300, bottom = 200; // Drawing the rectangle rectangle(left, top, right, bottom); getch(); closegraph(); return 0; } |
20
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
#include <stdio.h> int main() { int rows = 3; int i, j; for (i = 0; i < rows; i++) { // Print 'M' characters for (j = i; j < rows; j++) { printf("M"); } // Print 'I' characters for (j = 0; j <= i * 2; j++) { printf("I"); } // Print 'B' characters for (j = i; j < rows; j++) { printf("B"); } printf("\n"); } return 0; } |
Group D
21
C programming language provides a variety of string manipulation functions in the standard library <string.h>
. Here are some commonly used string library functions along with examples for each:
- strlen(): Returns the length of the string.
1 2 3 4 5 6 7 8 9 |
#include <stdio.h> #include <string.h> int main() { char str[] = "Hello, World!"; int length = strlen(str); printf("Length of the string: %d\n", length); return 0; } |
strcpy(): Copies one string to another.
1 2 3 4 5 6 7 8 9 10 |
#include <stdio.h> #include <string.h> int main() { char source[] = "Hello, World!"; char destination[20]; strcpy(destination, source); printf("Copied string: %s\n", destination); return 0; } |
strcat(): Concatenates two strings.
1 2 3 4 5 6 7 8 9 10 |
#include <stdio.h> #include <string.h> int main() { char str1[20] = "Hello"; char str2[] = " World!"; strcat(str1, str2); printf("Concatenated string: %s\n", str1); return 0; } |
strcmp(): Compares two strings.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
#include <stdio.h> #include <string.h> int main() { char str1[] = "Hello"; char str2[] = "Hello"; if (strcmp(str1, str2) == 0) { printf("Strings are equal.\n"); } else { printf("Strings are not equal.\n"); } return 0; } |
strchr(): Finds the first occurrence of a character in a string.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
#include <stdio.h> #include <string.h> int main() { char str[] = "Hello, World!"; char *ptr = strchr(str, 'o'); if (ptr != NULL) { printf("Character 'o' found at position: %ld\n", ptr - str + 1); } else { printf("Character not found.\n"); } return 0; } |
strstr(): Finds the first occurrence of a substring in a string.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
#include <stdio.h> #include <string.h> int main() { char str[] = "Hello, World!"; char *ptr = strstr(str, "World"); if (ptr != NULL) { printf("Substring 'World' found at position: %ld\n", ptr - str + 1); } else { printf("Substring not found.\n"); } return 0; } |
22.
Passing arguments by value and passing arguments by address are two different ways to pass parameters to functions in C, each with its advantages and disadvantages.
Passing Arguments by Value:
When passing arguments by value, the function receives a copy of the actual parameter’s value. Any modifications made to the parameter within the function do not affect the original variable in the calling function.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
#include <stdio.h> void increment(int x) { x++; printf("Inside function: %d\n", x); } int main() { int num = 5; printf("Before function call: %d\n", num); increment(num); printf("After function call: %d\n", num); return 0; } |
Passing Arguments by Address:
When passing arguments by address (also known as passing by reference), the function receives the address (memory location) of the actual parameter. This allows the function to directly modify the value of the variable in the calling function.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
#include <stdio.h> void increment(int *x) { (*x)++; printf("Inside function: %d\n", *x); } int main() { int num = 5; printf("Before function call: %d\n", num); increment(&num); printf("After function call: %d\n", num); return 0; } |